Vehicles, Obstacles, Walls¶
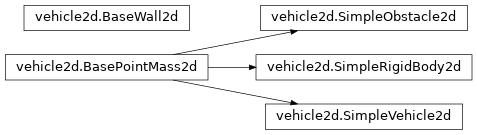
Wall, Obstacle, Pointmass, and Vehicle classes.
BaseWall2d: Static, one-directional wall segments.
BasePointMass2d: Point mass with heading aligned to velocity.
SimpleObstacle2d: Immobile obstacle with center and bounding radius.
SimpleVehicle2d: BasePointMass2d with attached Navigator for steering.
SimpleRigidBody2d: (Experimental) mass with basic rotational physics.
Rendering is done independently of this module, but for convenience each class provides a set_spriteclass() class method. Once this is set, the constructors above will use the optional spritedata parameter to pass through rendering information to the sprite class.
- class vehicle2d.BaseWall2d(center, length, thick, f_normal, spritedata=None)¶
A base class for static, wall-type obstacles.
- Parameters
Note
Walls are one-way due to the associated steering behaviours.
- classmethod set_spriteclass(spriteclass)¶
Set the default sprite class used to render this type of object.
- class vehicle2d.BasePointMass2d(position, velocity, radius, mass, maxspeed, maxforce, spritedata=None)¶
A moving object with rectilinear motion and optional sprite.
- Parameters
position (Point2d) – Center of mass.
velocity (Point2d) – Velocity vector (initial facing is aligned to this).
radius (float) – Bounding radius of the object.
mass (float) – Total mass.
maxspeed (float) – Maximum speed.
maxforce (float) – Maximum force available per update.
spritedata (optional) – Extra data for rendering; see module notes.
This provides a minimal base class for a 2D pointmass with bounding radius and heading aligned to velocity. Use move() for rectilinear physics updates each cycle (including applying force).
- classmethod set_spriteclass(spriteclass)¶
Set the default sprite class used to render this type of object.
- accumulate_force(force_vector)¶
Add a new force to what’s already been accumulated.
- Parameters
force_vector (Point2d) – Added to any previously-accumulated force.
This function is intended to allow multiple sources to exert force on an object without needing to compute those forces all at once. Use move() below with force_vector=None to apply the resultant force accumulated by this method and reset accumulated force to zero.
- move(delta_t=1.0, force_vector=None)¶
Updates rectilinear position, velocity, and acceleration.
- Parameters
delta_t (float) – Time increment since last move.
force_vector (Point2d, optional) – Vector force to apply during this update.
If force_vector is None (the default), we use the force accumulated by self.accumulate_force() since the last call to this method, and zero out the accumulated force. Otherwise, apply the force_vector given, and leave the accumulated force unaffected.
In any case, the maximum force and resulting velocity are limited by maxforce and maxspeed attributes.
- class vehicle2d.SimpleObstacle2d(position, facing, radius, spritedata=None)¶
A static obstacle with center and bounding radius.
- Parameters
- move(delta_t=1.0, force_vector=None)¶
Does nothing; SimpleObstacles are immovable.
- class vehicle2d.SimpleVehicle2d(position, velocity, radius, mass, maxspeed, maxforce, spritedata=None)¶
Point mass with attached Navigator for steering behaviours.
- Parameters
position (Point2d) – Center of mass.
velocity (Point2d) – Velocity vector (initial facing is aligned to this).
radius (float) – Bounding radius of the object.
mass (float) – Total mass.
maxspeed (float) – Maximum speed.
maxforce (float) – Maximum force available per update.
spritedata (optional) – Extra data for rendering; see module notes.
Use this class for basic steering.Navigator functionality. Calling move() will get the current steering force from the Navigator and apply it.
- move(delta_t=1.0, force_vector=None)¶
Compute steering force (via Navigator); update rectilinear motion.
- class vehicle2d.SimpleRigidBody2d(position, velocity, beta, omega, radius, mass, maxspeed, maxforce, inertia, maxomega, maxtorque, spritedata=None)¶
Moving object with linear and angular motion, with optional sprite.
- Parameters
position (Point2d) – Center of mass.
velocity (Point2d) – Velocity vector.
beta (float) – Offset angle between heading/velocity and facing.
omega (float) – Angular speed.
radius (float) – Bounding radius of the object.
mass (float) – Total mass.
maxspeed (float) – Maximum speed.
maxforce (float) – Maximum force available per update.
inertia (float) – Rotational inertia.
maxomega (float) – Maximum magnitude of angular speed.
maxtorque (float) – Maximum magnitude of torque per update.
spritedata (optional) – Extra data for rendering; see module notes.
Warning
Still experimental!
- move(delta_t=1.0, force_vector=None)¶
Updates position, velocity, and acceleration.
- Parameters
delta_t (float) – Time increment since last move.
force_vector (Point2d, optional) – Constant force during this update.
Note
We must override BasePointMass2d.move() in order to avoid aligning our heading with forward velocity.
- rotate(delta_t=1.0, torque=0)¶
Updates heading, angular velocity, and torque.
- Parameters
delta_t (float) – Time increment since last rotate.
torque (float, optional) – Constant torque during this update.